From Zero to Hero: A Complete Flutter Tutorial for Beginners
Flutter has become one of the most popular frameworks for mobile app development. It allows developers to create beautiful, high-performance applications for multiple platforms using a single codebase. If you are new to Flutter, this Flutter tutorial for beginners will help you understand the basics and get started with your first Flutter project.

Introduction
Flutter has become one of the most popular frameworks for mobile app development. It allows developers to create beautiful, high-performance applications for multiple platforms using a single codebase. If you are new to Flutter, this Flutter tutorial for beginners will help you understand the basics and get started with your first Flutter project.
What is Flutter?
Flutter is an open-source UI software development toolkit created by Google. It is used to build natively compiled applications for mobile, web, and desktop from a single codebase. Unlike traditional frameworks, Flutter provides a rich set of pre-designed widgets that help create responsive and visually appealing applications.
Key Features of Flutter
- Cross-platform development – Build apps for Android, iOS, web, and desktop with a single codebase.
- Fast development – With hot reload, changes appear instantly without restarting the app.
- Beautiful UI – Offers customizable widgets to create stunning user interfaces.
- High performance – Uses Dart programming language for efficient and smooth app performance.
Setting Up Flutter
Before you start, you need to set up your development environment. Follow these steps:
1. Install Flutter SDK
Download and install Flutter from the official website. Follow the installation guide for your operating system (Windows, macOS, or Linux).
2. Set Up an Editor
You can use any code editor, but Visual Studio Code and Android Studio are recommended. Install the Flutter and Dart plugins for a better development experience.
3. Check the Installation
Run the following command in the terminal to verify if Flutter is installed correctly:
sh
CopyEdit
flutter doctor
This will show a list of required dependencies and their installation status.
Understanding the Flutter Architecture
Flutter is based on a reactive framework and follows a widget-based approach. Here’s a basic overview:
- Widgets – Everything in Flutter is a widget, including buttons, text, and layout structures.
- State Management – Manages changes in the app’s UI, with various approaches like Provider, Riverpod, and Bloc.
- Dart Programming – Flutter uses Dart, an object-oriented language optimized for UI development.
Building Your First Flutter App
1. Create a New Flutter Project
Open your terminal or command prompt and run:
sh
CopyEdit
flutter create my_first_app
This will create a new Flutter project with a default template.
2. Run the App
Navigate to the project folder and run:
sh
CopyEdit
cd my_first_app
flutter run
This will launch a default counter app on your connected device or emulator.
Exploring Flutter Widgets
Widgets are the building blocks of a Flutter app. Some commonly used widgets include:
- Text Widget – Displays text in your app.
- Container Widget – A flexible box for layout styling.
- Column and Row Widgets – Used to arrange elements vertically or horizontally.
- Scaffold Widget – Provides a basic app structure, including an app bar, body, and floating button.
Example of a Simple Flutter UI
Here’s an example of how a basic UI is structured in Flutter:
dart
CopyEdit
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Flutter Tutorial')),
body: Center(child: Text('Hello, Flutter!')),
),
);
}
}
This code creates a simple app with a title bar and a centered text.
Flutter State Management
Managing state is crucial in Flutter apps. There are two types of state management:
- Stateless Widgets – Do not change over time (e.g., static UI elements).
- Stateful Widgets – Can change dynamically based on user interactions.
Example of a Stateful Widget
dart
CopyEdit
class CounterApp extends StatefulWidget {
@override
_CounterAppState createState() => _CounterAppState();
}
class _CounterAppState extends State<CounterApp> {
int _count = 0;
void _increment() {
setState(() {
_count++;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Counter App')),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text('Counter: $_count'),
ElevatedButton(
onPressed: _increment,
child: Text('Increase'),
),
],
),
),
);
}
}
This creates a button that updates the counter value when clicked.
Debugging and Testing
Flutter provides several debugging tools to help developers build efficient apps:
- Debug Mode – Identifies UI issues and performance bottlenecks.
- Flutter DevTools – Offers a visual interface for debugging layouts and network requests.
- Unit Testing – Ensures app components work correctly.
To run tests, use:
sh
CopyEdit
flutter test
Deploying Your Flutter App
Once your app is ready, you can deploy it to the Play Store or App Store.
Steps to Deploy:
- Build the APK for Android
sh
CopyEdit
flutter build apk
- Build for iOS
sh
CopyEdit
flutter build ios
Upload the generated files to the respective stores following their guidelines.
Conclusion
This Flutter tutorial covered the fundamentals of Flutter, from setup to building and deploying an app. By learning the core concepts, you can start developing your own applications. Keep practicing, experiment with different widgets, and explore state management techniques to enhance your skills.
Flutter provides endless possibilities for app development, making it an excellent choice for beginners and experienced developers alike. Start your Flutter journey today and turn your ideas into powerful mobile applications! ????
What's Your Reaction?




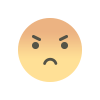

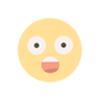